This example showcases how a Laravel application can utilize the ldap-record package for user authentication against an Active Directory (AD) server. Here’s a breakdown:
Configuration Settings:
The following code consists of the configuration settings of a .env file to establish a connection with the AD server:
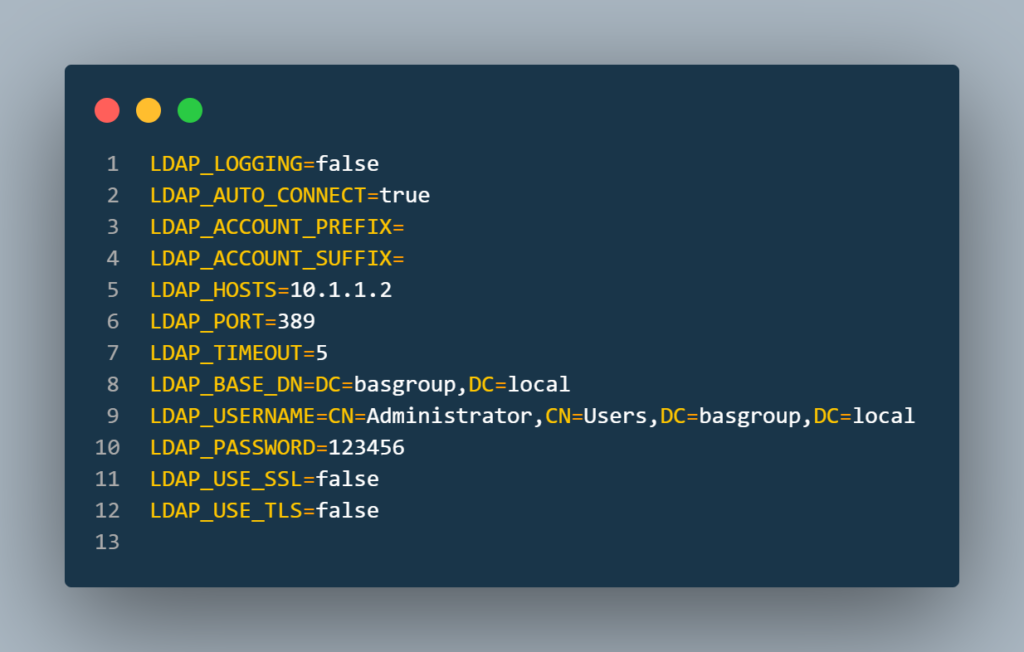
Logging & Connection:
- LDAP_LOGGING: Dictates if LDAP operations should be logged.
- LDAP_AUTO_CONNECT: Specifies if a connection to the AD server should be established immediately upon application boot.
Connection Details:
- LDAP_HOSTS, LDAP_PORT, etc.: These define the necessary parameters like the IP address of the AD server, port, base distinguished name, and the credentials/bind account(username and password).
Security Protocols:
- LDAP_USE_SSL & LDAP_USE_TLS: Flags to determine if SSL or TLS security protocols should be used for the connection.
LDAP Providers & Connections:
This section defines the connection and provider details:
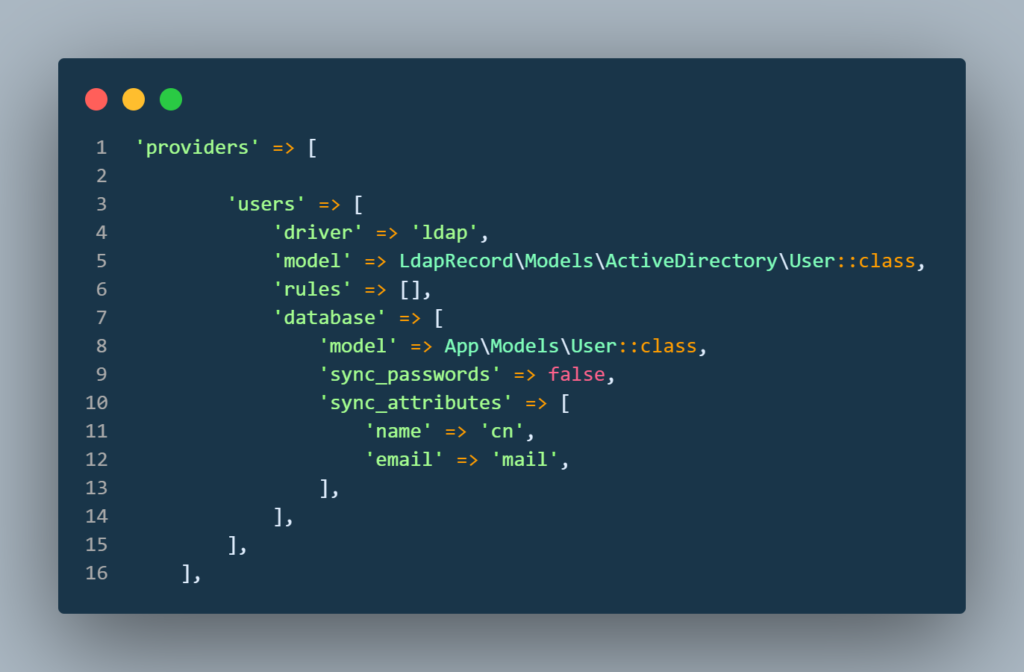
Providers:
- ‘driver’: Specifies that the authentication driver in use is LDAP.
- ‘model’: Points to the Active Directory user model in the ldap-record package.
- ‘database’: Defines the local Laravel application user model, how the data should sync, and maps the AD attributes (cn and mail) to the local user model attributes (name and email).
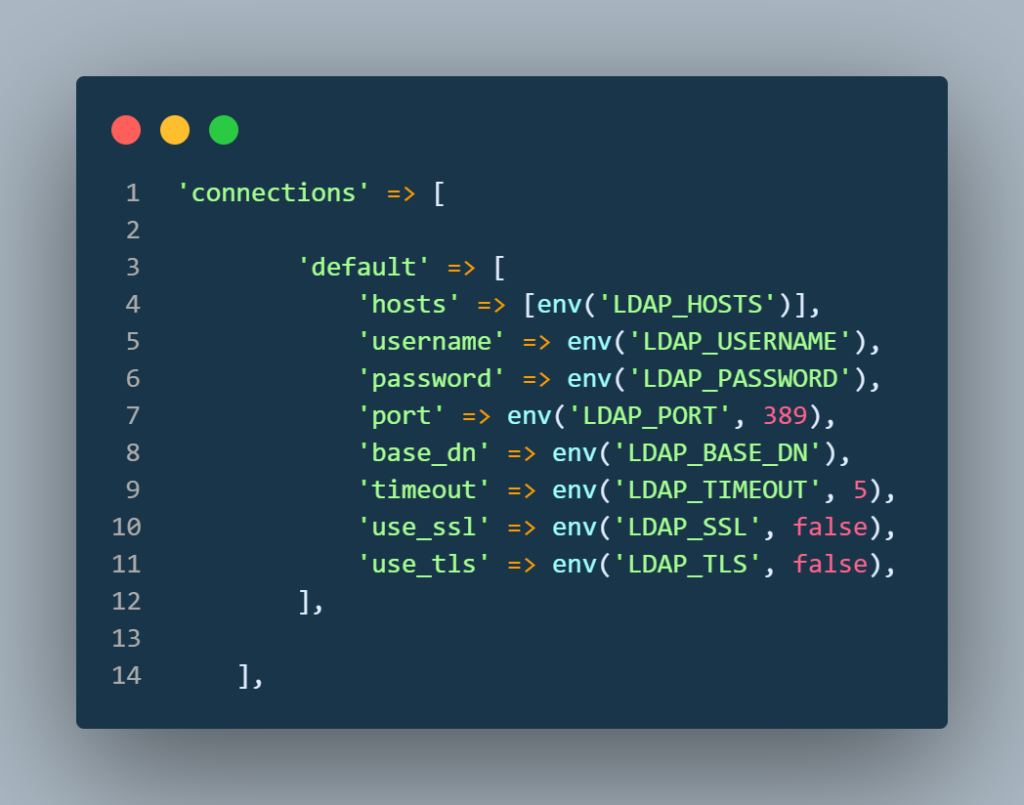
Connections:
- ‘default’: The default connection array pulls the configuration settings (like hosts, username, password, etc.) from the environment variables.
Authentication Function:
The authenticate function manages the actual process of authenticating a user:

Main Authentication Method:
- authenticate(Request $request): This method kicks off the process. It gets the email input from the request, which is used as a username to search for the user in the LDAP directory.
- The method then checks whether this user exists in LDAP and proceeds to synchronize the LDAP user’s data with the local database.
- After that, it fetches the contact data and adds it to the user model.
- Finally, the user is logged into the application and redirected to the home page. If no LDAP user is found, an error message is returned.
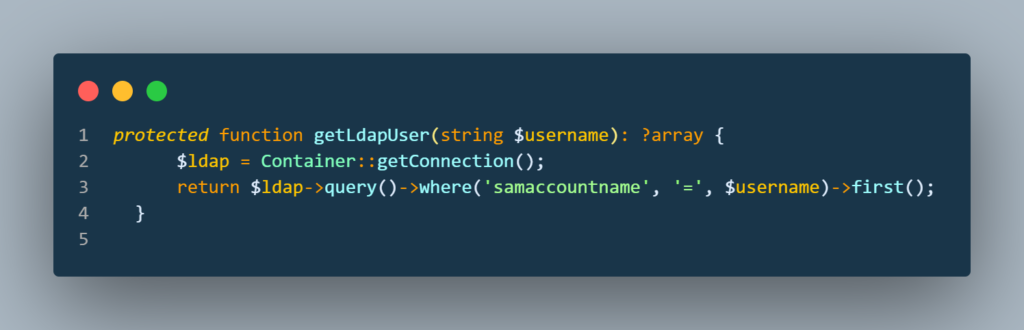
Retrieve the LDAP User:
- getLdapUser(string $username): This method interacts with the LDAP server. It creates a query to search for a user with the provided username. If found, it returns the LDAP user’s attributes, and if not, it returns null.
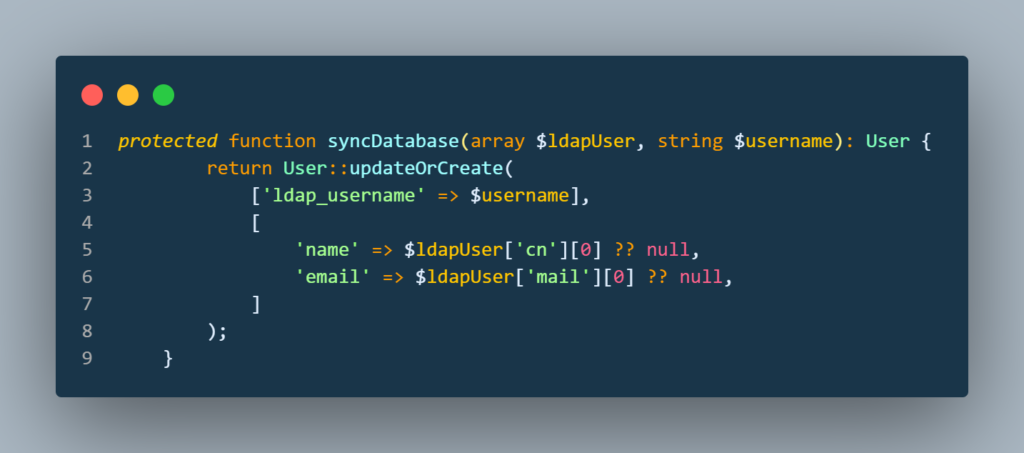
Synchronize LDAP with Local Database:
- syncDatabase(array $ldapUser, string $username): This method is used to create or update the user record in the local Laravel database with the data retrieved from the LDAP server. If a user with the given LDAP username exists, the database is updated. Otherwise, a new record is created.
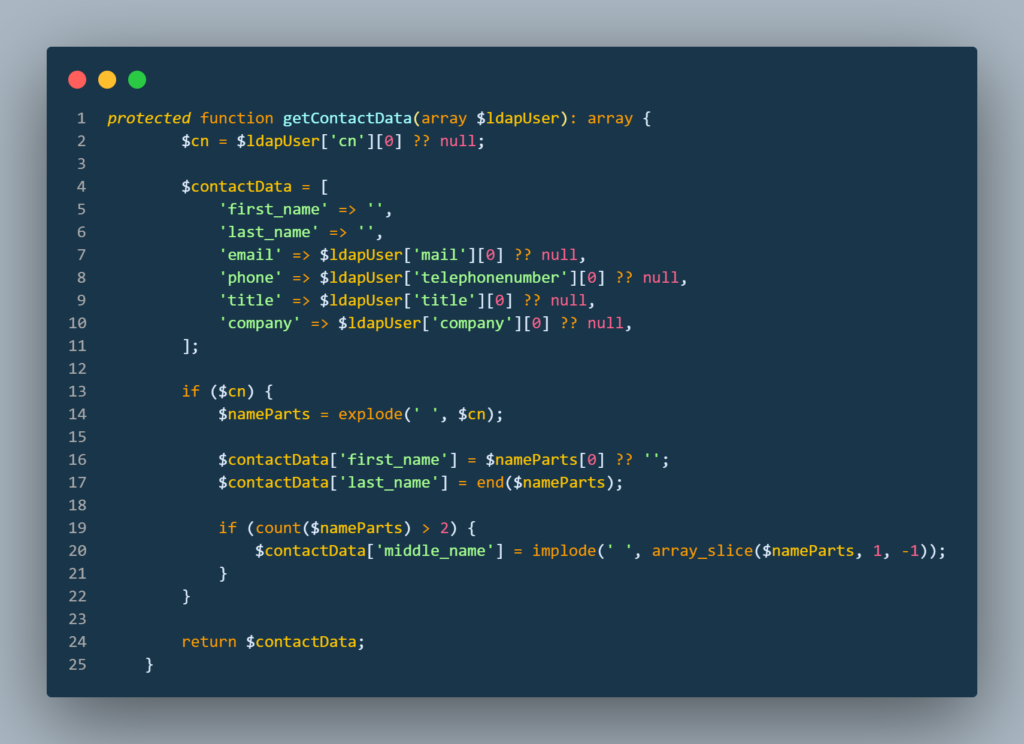
Extract Contact Data from LDAP Attributes:
- getContactData(array $ldapUser): This method processes the LDAP user attributes and extracts contact details such as first_name, last_name, email, phone, title, and company. It also contains logic to split the full name (cn) into first, middle, and last names, catering for various name structures.
Summary
By leveraging the ldap-record package, the example offers a seamless bridge between AD and the application, enabling users to authenticate with their AD credentials while also synchronizing user data between the two systems. If you would like to incorporate your AD server into your next project, feel free to reach out or visit the ldap-record site for more details and customization options.